DXStudio AddOns Collection
Quote: “DX Studio is a 3D editing + engine suite for Windows XP/Vista/7/8/10. It was most popular between 2005 and 2010, but can still be a useful tool for creating interactive 2D+3D on Windows.”
This is a collection of addons I made for DXStudio – back then. Might be of limited use nowadays…
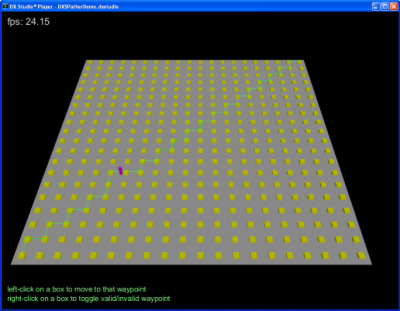
DXSPather Dll
DXSPather is a C++ A* pathfinding DLL that can be used with DXStudio documents (and hopefully gives some speed advantages over doing all the calculation in JS script). It comes with a javascript include file that you would add to your project. This script then provides “proxy functions” that in turn interact with the dll and handle path setup and calculation.
The DLL utilizes a ready-made C-class for A* handling, done by Lee Thomason (www.grinninglizard.com). It is named “micropather”, and fast and rather easy to use. If you’d ever need some C-code for pathfinding, that thing is the way to go, imho.
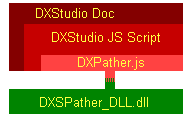
Features
This implementation of the DLL executes the calculation of a certain path request within one call. For rather large maps (waypoint numbers), this may cause a single “hickup” condition in the DXStudio doc.
The waypoint attribute buffer (not the waypoints themselves) is dynamic, so you can make single waypoints “valid” or “inaccessible” by calling functions, and these conditions will be considered at the very next waypoint calculation request.
Allowed movement directions are adjustable, too. So, you could e.g. choose to have a path calculated only by moving “diagonal” (X+/-1 AND Y+/-1) and “left-right” (X+/-1 ONLY), but not “up-down” (Y+/-1 ONLY), which could be useful for hexagon maps.
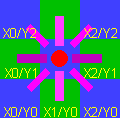
Exposed Wrapper Functions
DXStudio users would not call DLL functions directly, but use “wrappers” provided in DXSPather.js. Note that waypoints (that you need to submit to the DLL once) need to be in regular grid order (e.g., in a regular [X,Y] indices format). Not necessarily do the waypoints’ distances need to be evenly spaced.
Functions and variables provided in the js include file are:
function DXSP_SetDllLocation(string sPathToDll)
(added with v1.3) Set the path to the dll inside the wrapper script. Usually, this would be something like system.folders.document. No return value.
function DXSP_SetDiagonalMovements(bool bMoveDiagonal)
(name changed with v1.2) Tells the path calculator if it should consider diagonal movements (X and Y index both change). Switching is possible by this function (changes will be valid on the very next path calculation). Returns always true.
function DXSP_SetUpDownMovements(bool bMoveUpDown)
(added with v1.2) Tells the path calculator if it should consider vertical movements (Y index changes only). This could be useful when processing paths on hexagon grid maps. Switching is possible by using this function (changes will be valid on the very next path calculation). Returns always true.
function DXSP_SetLeftRightMovements(bool bMoveLeftRight)
(added with v1.2) Tells the path calculator if it should consider horizontal movements (X index changes only). This could be useful when processing paths on hexagon grid maps. Switching is possible by using this function (changes will be valid on the very next path calculation). Returns always true.
function DXSP_SetMapSize(int iX, iY)
This call resets the DLL data and prepares a (yet empty) X*Y waypoint grid to be filled later on. Returns false on error, true on success.
function DXSP_SetMapNodeData(int iIndexX, iIndexY, float fPosX, fPosY, fPosZ)
Sets the basic data of one waypoint “node”. Submit its indices in the (regular grid’s) waypoint array (iIndexX, iIndexY), as well as its positions in 3D space (fPosX, fPosY, fPosZ). This data has to be submitted once before any path calculation requests, and remains stored inside the DLL. Returns false on error, true on success.
function DXSP_SetMapNodeCostModifier(int iIndexX, iIndexY, float fCostModifier)
(added with v1.3, EXPERIMENTAL) Sets an optional factor for a waypoint that will be considered when calculating paths. Use this to make certain waypoints more or less “desireable” for a path. E.g., a fCostModifier of 2.0 would make a waypoint more “uneasy”, while a modifier of “0.5” would make this waypoint being prefered. By default, the cost modifier for each waypoint is “1.0f” (which is a neutral factor). Experimental feature. Setting values here might cause weird paths to be created.
function DXSP_SetMapNodeWalkable(int iIndexX, iIndexY, bool bWalkable)
Makes a certain waypoint “walkable” (being considered in path calculations) – or disables it. Data can be dynamically changed (read: whenever you need), and will be consided at the very next path calculation. Returns false on error, true on success.
function DXSP_SolvePath(int iIndexXFrom, iIndexYFrom, iIndexXTo, iIndexYTo)
This routine initiates a path calculation, taking the “from” and “to” waypoint indices as arguments. As soon as this call returns, the calculated path is available in (global) variables inside the include js script (see below).
Array DXSP_WaypointsX
Array DXSP_WaypointsY
As soon as a call to DXSP_SolvePath succeeded, these two array variables contain the (X,Y) indices of the waypoints to travel (including start/end waypoint). Access would be possible by e.g. system.script.DXSP_WaypointsX[0] or system.script.DXSP_WaypointsX.length
Downloads
The download comes with a DXStudio demo doc, where you can select a path of an object. The available waypoints are shown as boxes.
File | Date | Size | Remarks |
DXPather_1.3.zip | 10/10/2009 | 255 KB | DXPather Package 1.3. The DXPather_DLL.dll, DXSPather.js and DXSPatherDemo_1.3.dxstudio |
DXSCheckMem Dll
DXSCheckMem is a DLL I once wrote to track dynamic memory usage changes of DXStudio documents. It can be called on a per-frame basis from JS script, and adds only little overhead to the fps (if at all).
Calling the DLL from DXStudio script
The DLL exposes one function only. Preferably, place it in the doc’s directory and add code like this to e.g. a 2D text object’s onUpdate function:
var mem = 0; function onUpdate() { mem = system.callDLL(system.folders.document+"dxs_chkmem.dll", "dxsChkMem", ""); mem = mem/1000; object.text = "Memory usage reported by dll: " + mem.toFixed(2) + " KBytes"; }
Downloads
File | Date | Size | Remarks |
DXSCeckMem_1.0.zip | 10/9/2009 | 1.5 MB | DXSCheckMem 1.0. The dxs_chkmem.dll and mem_dll_test.dxstudio |
(This is a Carrara render. Not ingame footage)
DXStudio Cannon Module
This is my first try to create a re-usable module for Worldweavers DXStudio. It is a laser cannon with targetting functions, some visual effects and sound. As for it’s quality, it might not completely fit your needs or standards out-of-the-box (especially for the texturing), but could maybe work as your base for own modules (I hope).
Features
Given a target 3D coordinate, the cannon will automatically align to this target, playing some mechanical sound. Speed of rotations can be adjusted. If requested, a laser beam will be initiated after alignment has completed. Automatically, there is a check if some scene object is hit. If so, an explosion animation will be started, accompanied by a corresponding sound. In case a scene object has been hit, a notify message is sent to the parent scene.
Restrictions
The module (‘object’) must not be rotated upon placing. It does only work when positioned on the XZ-plane. Different heights (Y) do work, though. It can be scaled in the scene editor. The cannon will not target positions that are too near (e.g., touching its own bounding box) or can not be aligned by the cannon tube (e.g., too low in y dimension). If a shot is being executed, alignment to new target positions will take place after having finished the shot.
Usage
Exposed module functions:
function cannonInitialize(id)
This must be called before using the module. Pass the module’s id (e.g. ‘objects.CannonModule_1.id’) as parameter.
function cannonSetRange(fBeamMaxRange, fBeamDuration)
Set the maximum range the laser beam can shoot (that is the range a hit detection will be done – and the length of the beam if nothing is hit) and the duration of a shot (in seconds).
function cannonSetSpeed(fTurnSpeed, fFocusSpeed)
With this, set the turn speed and the speed of the cannon tube’s up/down movement (deg/sec). Used for alignment.
function cannonSetNewTargetPosition(vTargetPosition)
Set a new 3D coordinate target position. The cannon will align to that, but not shoot. No movement will happen if the target position can not be aligned to.
function cannonSetNewTargetPositionAndShoot(vTargetPosition)
Same as before, but the cannon will initialize a laser shot after having focused the target coordinates.
function cannonStopMovement()
Stop a currently running alignment movement (if any).
function cannonMovementRunning()
Returns true if the cannon is in the process of alignment to a given target coordinate. False, if not.
function cannonShoot()
Initiate a laser shot. Will stop any alignment movement immediately, if present.
Whenever a hit is detected, scene.moduleNotify(msg) of the parent scene will be called. Passing TYPE of the event (=CM aka CannonModule), ID of the module and the beam hit position TARGET. Possible target objects must have their selectionMask set to 2 (bit1 = 1). Use ‘eval()’ in moduleNotify function.
Please see the .dxstudio demo program for a detailed usage demo.
Downloads
File | Date | Size | Remarks |
Cannonmodule_Demo.exe.zip | 12/31/2008 | 10 MB | A demo exe, incorporationg the cannon module |
Test_Cannonmodule.dxstudio.zip | 12/31/2008 | 741 KB | The source of the demo exe as a dxstudio scene file |
CannonModule.dxmodule.zip | 12/31/2008 | 463 KB | The cannon module |
Scene_Cannonmodule.dxstudio.zip | 12/31/2008 | 463 KB | The cannon module as a dxstudio scene file. Just for convenience |
Cannon_Models_and_Textures.zip | 12/31/2008 | 490 KB | The model’s textures and parts, in case you want to fiddle yourself. Includes textures (bmp) and skinned models (fbx and .x) |